UPDATE: As of Xcode 3.2 you can rename projects from the Projects->Rename dropdown.
UPDATE: Be sure to see my post about the shell script I wrote to rename from the command line.
For those wanting to rename their XCode project, here how you do it manually:
1) copy your project to a new folder, rename the folder to your new project name, delete the build directory in the new folder.
2) drag the entire new project folder onto the TextMate text editor
3) In TextMate, remove (delete references) to anything non-text such as images and sounds. [UPDATE: TextMate will ignore the non-text files, so step 3 is unnecessary.]
4) Replace in Project (old project name) with (new project name), save all files. Close TextMate.
5) Now drag the (project name).xcodeproj file into Text Mate, repeat Replace in Project processm, save all files. Close Text Mate. If you have spaces in your project name, look for all instances of your project with spaces replaced with underscores too.
6) Rename all files containing (old project name) to (new project name). Be sure to check the Classes directory too. If your project name contains spaces, look for all instances of filenames with spaces replaced with underscores too.
That's it! Your new project should be good to go. I don't think cleaning of targets is necessary, since the build directory is removed (correct me if I'm wrong though!)
Wednesday, December 17, 2008
Tuesday, December 9, 2008
iDVD with a PC burner
So you have a Mac with iDVD, but no superdrive (DVD burner.) However, you have this trusty old PC with a DVD burner. Wouldn't it be nice to burn your iDVD projects on the PC's burner? Well you can, and here is how:
1) Once your iDVD project is ready for burning, go to File->Save as disc image. This will save the project as an .img file.
2) In the finder, double click the .img file. This will mount the image.
3) Open Disc Utility. Click on your mounted image on the left pane and click "Convert". Choose the "CD Master .cdr" option. This will create a .cdr file.
4) Copy the .cdr file to a place where you can access it from your PC. Rename *.cdr to *.iso.
5) On the PC, Use your favorite DVD burner software to burn the .iso image to a DVD. I like ImgBurn, it's free and easy to use.
That's it, hope that helps!
1) Once your iDVD project is ready for burning, go to File->Save as disc image. This will save the project as an .img file.
2) In the finder, double click the .img file. This will mount the image.
3) Open Disc Utility. Click on your mounted image on the left pane and click "Convert". Choose the "CD Master .cdr" option. This will create a .cdr file.
4) Copy the .cdr file to a place where you can access it from your PC. Rename *.cdr to *.iso.
5) On the PC, Use your favorite DVD burner software to burn the .iso image to a DVD. I like ImgBurn, it's free and easy to use.
That's it, hope that helps!
Thursday, November 6, 2008
New Mac Mini to combine features of AppleTV?
So what is to become of the new mac mini? Some say it's dead, but I think it's way too purposeful to kill it. In many ways, it duplicates the functionality of the apple tv, so it may become a combination of the two: A small mac computer with HDMI (or MiniDP.) Couple that with the slimmer "brick" aluminum case design, the new video capabilities and speed of the current mackbook, and you have a pretty hellish HTPC. No Blu-ray for sure, it is counter productive in both cost and purpose. Maybe get rid of the optical drive altogether, and go with air drives and streaming content. Your thoughts?
Thursday, October 30, 2008
Chances of quad aces against royal flush?
In the WSOP 2008, a royal flush beat out quad aces. What are the chances of that happening? I know they gave a result and I'm not sure how that was calculated, but I did my own calculation with my own handy online odds calculator. Let me know if I screwed it up :)
Here is a link to the calculation:
link
I'll step through the process I took:
The first player needs pocket aces. So of the 52 cards, we have 4 outs, we need 2, and we are drawing 2 cards.
For the second player, first he needs any one of the KQJT that is not the same suit of the other players hole cards. 50 cards left, there are 8 outs in the deck, we are drawing 1 card and we need 1 of the outs.
For the other hole card , we need one of the remaining KQJT of the same suit. 49 cards left, there are 3 outs, we are drawing 1 and need 1.
On the board (don't care what order or where), we need exactly 4 outs from the 5 cards: the other two aces, and the other two cards KQJT of the same suit. 48 cards left, drawing 5, 4 outs, we need all 4.
Answer, this happens in 877,963,124:1, or 0.000001139% of the time.
I think they came up with 2.x billion? I'm curious how they figured it.
Here is a link to the calculation:
link
I'll step through the process I took:
The first player needs pocket aces. So of the 52 cards, we have 4 outs, we need 2, and we are drawing 2 cards.
For the second player, first he needs any one of the KQJT that is not the same suit of the other players hole cards. 50 cards left, there are 8 outs in the deck, we are drawing 1 card and we need 1 of the outs.
For the other hole card , we need one of the remaining KQJT of the same suit. 49 cards left, there are 3 outs, we are drawing 1 and need 1.
On the board (don't care what order or where), we need exactly 4 outs from the 5 cards: the other two aces, and the other two cards KQJT of the same suit. 48 cards left, drawing 5, 4 outs, we need all 4.
Answer, this happens in 877,963,124:1, or 0.000001139% of the time.
I think they came up with 2.x billion? I'm curious how they figured it.
Tuesday, October 28, 2008
Getting URL from UIWebView
It thought I'd post how to get the current URL string from a UIWebView object. Here you go:
That is assuming myWebView is your UIWebView object.
To open a web page in Safari from a UIWebView, do this:
NSString *currentURL
= myWebView.request.URL.absoluteString;
That is assuming myWebView is your UIWebView object.
To open a web page in Safari from a UIWebView, do this:
[[UIApplication sharedApplication]
openURL:myWebView.request.URL];
Friday, October 17, 2008
Thursday, October 16, 2008
Objective-C Crash Course for PHP developers
I've been dabbling around in Objective-C lately, and I thought I'd share some of my experiences with it. It took some time to get my head around a lot of the basic programming syntax. I thought I'd share some things I've learned, and how it compares to PHP.
First and foremost, Objective-C is a compiled language, whereas PHP is a scripted language. Objective-C requires compiling into a binary before it is executed. There is no need to compile PHP, as the script is interpreted at run-time.
PHP was once strictly a procedural language, but over time many object-oriented language features have been added. Objective-C is an Object-Oriented programming language structure that is built on top of C, which is a procedural language. You can freely mix C with Objective-C (as well as C++), much the same that you can mix PHP procedural functions with PHP objects and classes.
Objective-C is foremost an Object-Oriented language. That means, everything is an object. Even a simple string is an object of NSString. And yes, you can mix C code and use plain procedural functions if you wish. Normally you can do everything you need with objects.
First, a "Hello World" application in PHP, then the same one in Objective-C.
The PHP version:
The Objective-C version:
HelloWorld.h header file:
HelloWorld.m implementation file:
main.m file:
If you have had any experience programming in C, much of this will look familiar. Objective-C is after all, just an extension of the C programming language, adding in all the OO goodness of Objective-C to the already existing C syntax. I'm going to refer to Objective-C as OC from here on out.
Notice that comments are identical in PHP, OC, and C. // for single line comments, and /* */ for multi-line comments. Easy enough. Now, lets take a look at the OC code, starting with the header file:
#import is much the same as include_once() in PHP. If a file gets imported more than once, it is ignored.
OC is made up of header files (.h) and source files (.m) Typically, each header file is paired with a source file. The header file declares the instance variables and methods of your classes, whereas the methods are implemented in the source (.m) file. You need only import the .h file, as the .m file is compiled by XCode automatically.
Foundation.h is an existing OC framework of classes. It includes much of the fundamental objects you will want when developing Objective-C. There are many other frameworks to choose from too. The core of PHP is procedural code, so there is no "foundation" to include. All of the basic PHP functionality is available at the outset of any PHP script (excluding special extensions and libraries of your own.)
The @interface line describes the name of your class, and also describes what object it is inherited from. Here we have a class named "HelloWorld" and it inherits NSObject, which is the most basic of objects. It is the top object of the inheritance tree. In PHP, there is no need to declare what object an non-extended class is inherited from.
In the curly braces of the @interface line, you declare what instance variables your class will contain. In our example, we have none declared.
After the curly brace and before the @end symbol, you declare your class methods, and the arguments they may take. The minus "-" at the beginning of the line declares that this is an instance method. If it began with a "+" it would be a class method. In PHP the "+" methods would be referred to as static methods. That is, a method called directly from the class, not from an object instance.
(void) is the value that is returned from the method. This method does not return a value.
@end defines the end of the method declarations.
And now the implementation (.m) file:
We import our HelloWorld.h header file.
Between the @implementation and @end lines, we implement the methods of our class.
This is an instance method, denoted by the "-" at the beginning. A "+" would have denoted a class method, or "static" method in PHP jargon. This method does not return anything, so (void) is used as the return declaration.
This is the body of our method. NSLog is the OC equivalent to the PHP's and C's printf function. %@ is a placeholder for our string object. NSCalendarDate is a class from the Foundation.h framework, and we are calling the calendarDate method of the class. It will return a string object containing a date for our NSLog.
This marks the end of our implementation methods.
And now for the main.m file:
Here we import our Foundation.h file. (Note we could have left this out, since the HelloWorld.h file imports it already.) Then we import the header file of our HelloWorld class.
As in C, every OC program must have a main() function. This is where the compiled binary will begin its execution, passing in any arguments given from the command line. In PHP, arguments to a script are captured in the $argv variable.
This is part of the memory management of OC. Every object in OC retains a count of how many references are attached to it. When an object is first instantiated, a reference count of 1 is placed on the object. Each time the object is retained, its reference count is incremented. Each time it is released, it is decremented. Once the ref count reaches zero, the object is destroyed. The autorelease pool is an OC convention used for memory management. When you autorelease an object (as opposed to just releasing it), it gets added to the pool of objects to be released at a later time. This way if something else needs to pick up your object before it is destroyed, it can, and you don't have to remember to release it again. OC 2.0 also has garbage collection features that help automate the tedious task of memory management. In PHP, memory management is automagically handled for you.
This is where your object is instantiated. [HelloWorld alloc] means we are calling the static method "alloc" of the HelloWorld class (a special method available to all OC classes.) alloc does the memory allocation for your object. Then, the init method is called of the instantiated object. This is much like the __construct() method of PHP objects, where initialization is handled, as well as initialization of any superclasses. The object is then given a pointer (of type HelloWorld) named *hw. Now we reference our object with hw from here onward. Note that in OC all objects require pointers to reference them.
Here we hand our object to the autorelease pool. Now I don't have to remember to release it later, and it's still available for me to use. The autorelease pool is kind of pointless for our HelloWorld app, but since the autorelease pool is an integral part of OC programming, I left it in. (I could have just did [hw release] after I was done with it.)
This is where we call the printHelloDate method of our class. In OC jargon, they call this a "message". I don't know exactly why, it's just calling a method of the object. When you see [object message] in OC, that is exactly what it is. The object is on the left, the message (method name) is on the right.
While we're on the subject of calling methods, You may see methods with parameters, but in OC they are named. Here is an example:
PHP:
Objective-C:
Here you see exactly what the parameters are for. In PHP I may have guessed that the first two params were X and Y, but the third would be a mystery without digging into the class. In OC, I can plainly see the first arg is X, the second is Y, and the third is setting the visiblity. Also in OC, you will typically see YES and NO used for booleans, not true and false.
One other tidbit: in Objective-C, the parameter names are part of the method name. So in PHP, the above method name is setSize(), whereas in Objective-C, the method name is setX:Y:visible.
And here we are releasing the autorelease pool, which will inevitably clean up our object from memory.
Our main function is expecting an integer to be returned, so we'll return a zero.
That's about it. If there are errors, send me a comment and I'll get them corrected. Thanks!
First and foremost, Objective-C is a compiled language, whereas PHP is a scripted language. Objective-C requires compiling into a binary before it is executed. There is no need to compile PHP, as the script is interpreted at run-time.
PHP was once strictly a procedural language, but over time many object-oriented language features have been added. Objective-C is an Object-Oriented programming language structure that is built on top of C, which is a procedural language. You can freely mix C with Objective-C (as well as C++), much the same that you can mix PHP procedural functions with PHP objects and classes.
Objective-C is foremost an Object-Oriented language. That means, everything is an object. Even a simple string is an object of NSString. And yes, you can mix C code and use plain procedural functions if you wish. Normally you can do everything you need with objects.
First, a "Hello World" application in PHP, then the same one in Objective-C.
The PHP version:
// HelloWorld.class.php
class HelloWorld
{
// instance variables go here
// methods go here
public function printHelloDate()
{
printf("Hello World! at %s",
strftime('%Y-%m-%d %H:%M:S %Z'));
}
}
// hello.php
include_once('HelloWorld.class.php');
$hw = new HelloWorld;
$hw->printHelloDate;
The Objective-C version:
HelloWorld.h header file:
#import <Foundation/Foundation.h>
@interface HelloWorld : NSObject {
// instance vars go here
}
// class methods go here
- (void)printHelloDate;
@end
HelloWorld.m implementation file:
#import "HelloWorld.h"
@implementation HelloWorld
- (void)printHelloDate
{
NSLog(@"Hello world! at %@",
[NSCalendarDate calendarDate]);
}
@end
main.m file:
#import <Foundation/Foundation.h>
#import "HelloWorld.h"
int main (int argc, const char * argv[]) {
NSAutoreleasePool * pool =
[[NSAutoreleasePool alloc] init];
HelloWorld *hw = [[HelloWorld alloc] init];
[hw autorelease];
[hw printHelloDate];
[pool release];
return 0;
}
If you have had any experience programming in C, much of this will look familiar. Objective-C is after all, just an extension of the C programming language, adding in all the OO goodness of Objective-C to the already existing C syntax. I'm going to refer to Objective-C as OC from here on out.
Notice that comments are identical in PHP, OC, and C. // for single line comments, and /* */ for multi-line comments. Easy enough. Now, lets take a look at the OC code, starting with the header file:
#import <Foundation/Foundation.h>
#import is much the same as include_once() in PHP. If a file gets imported more than once, it is ignored.
OC is made up of header files (.h) and source files (.m) Typically, each header file is paired with a source file. The header file declares the instance variables and methods of your classes, whereas the methods are implemented in the source (.m) file. You need only import the .h file, as the .m file is compiled by XCode automatically.
Foundation.h is an existing OC framework of classes. It includes much of the fundamental objects you will want when developing Objective-C. There are many other frameworks to choose from too. The core of PHP is procedural code, so there is no "foundation" to include. All of the basic PHP functionality is available at the outset of any PHP script (excluding special extensions and libraries of your own.)
@interface HelloWorld : NSObject {
// instance vars go here
}
The @interface line describes the name of your class, and also describes what object it is inherited from. Here we have a class named "HelloWorld" and it inherits NSObject, which is the most basic of objects. It is the top object of the inheritance tree. In PHP, there is no need to declare what object an non-extended class is inherited from.
In the curly braces of the @interface line, you declare what instance variables your class will contain. In our example, we have none declared.
// class methods go here
- (void)printHelloDate;
@end
After the curly brace and before the @end symbol, you declare your class methods, and the arguments they may take. The minus "-" at the beginning of the line declares that this is an instance method. If it began with a "+" it would be a class method. In PHP the "+" methods would be referred to as static methods. That is, a method called directly from the class, not from an object instance.
(void) is the value that is returned from the method. This method does not return a value.
@end defines the end of the method declarations.
And now the implementation (.m) file:
#import "HelloWorld.h"
We import our HelloWorld.h header file.
@implementation HelloWorld
Between the @implementation and @end lines, we implement the methods of our class.
- (void)printHelloDate
This is an instance method, denoted by the "-" at the beginning. A "+" would have denoted a class method, or "static" method in PHP jargon. This method does not return anything, so (void) is used as the return declaration.
{
NSLog(@"Hello world! at %@",
[NSCalendarDate calendarDate]);
}
This is the body of our method. NSLog is the OC equivalent to the PHP's and C's printf function. %@ is a placeholder for our string object. NSCalendarDate is a class from the Foundation.h framework, and we are calling the calendarDate method of the class. It will return a string object containing a date for our NSLog.
@end
This marks the end of our implementation methods.
And now for the main.m file:
#import <Foundation/Foundation.h>
#import "HelloWorld.h"
Here we import our Foundation.h file. (Note we could have left this out, since the HelloWorld.h file imports it already.) Then we import the header file of our HelloWorld class.
int main (int argc, const char * argv[]) {
As in C, every OC program must have a main() function. This is where the compiled binary will begin its execution, passing in any arguments given from the command line. In PHP, arguments to a script are captured in the $argv variable.
NSAutoreleasePool * pool =
[[NSAutoreleasePool alloc] init];
This is part of the memory management of OC. Every object in OC retains a count of how many references are attached to it. When an object is first instantiated, a reference count of 1 is placed on the object. Each time the object is retained, its reference count is incremented. Each time it is released, it is decremented. Once the ref count reaches zero, the object is destroyed. The autorelease pool is an OC convention used for memory management. When you autorelease an object (as opposed to just releasing it), it gets added to the pool of objects to be released at a later time. This way if something else needs to pick up your object before it is destroyed, it can, and you don't have to remember to release it again. OC 2.0 also has garbage collection features that help automate the tedious task of memory management. In PHP, memory management is automagically handled for you.
HelloWorld *hw = [[HelloWorld alloc] init];
This is where your object is instantiated. [HelloWorld alloc] means we are calling the static method "alloc" of the HelloWorld class (a special method available to all OC classes.) alloc does the memory allocation for your object. Then, the init method is called of the instantiated object. This is much like the __construct() method of PHP objects, where initialization is handled, as well as initialization of any superclasses. The object is then given a pointer (of type HelloWorld) named *hw. Now we reference our object with hw from here onward. Note that in OC all objects require pointers to reference them.
[hw autorelease];
Here we hand our object to the autorelease pool. Now I don't have to remember to release it later, and it's still available for me to use. The autorelease pool is kind of pointless for our HelloWorld app, but since the autorelease pool is an integral part of OC programming, I left it in. (I could have just did [hw release] after I was done with it.)
[hw printHelloDate];
This is where we call the printHelloDate method of our class. In OC jargon, they call this a "message". I don't know exactly why, it's just calling a method of the object. When you see [object message] in OC, that is exactly what it is. The object is on the left, the message (method name) is on the right.
While we're on the subject of calling methods, You may see methods with parameters, but in OC they are named. Here is an example:
PHP:
$rectangle->setSize(10,20,true);
Objective-C:
[rectangle setX:10 Y:20 visible:YES]
Here you see exactly what the parameters are for. In PHP I may have guessed that the first two params were X and Y, but the third would be a mystery without digging into the class. In OC, I can plainly see the first arg is X, the second is Y, and the third is setting the visiblity. Also in OC, you will typically see YES and NO used for booleans, not true and false.
One other tidbit: in Objective-C, the parameter names are part of the method name. So in PHP, the above method name is setSize(), whereas in Objective-C, the method name is setX:Y:visible.
[pool release];
And here we are releasing the autorelease pool, which will inevitably clean up our object from memory.
return 0;
Our main function is expecting an integer to be returned, so we'll return a zero.
That's about it. If there are errors, send me a comment and I'll get them corrected. Thanks!
Tuesday, October 14, 2008
Sharing speakers with Windows XP
I have a mac mini that I want to share the computer speakers on my PC with. No problem, just run a 1/8 inch male-male stereo cable from the line-out (headphone jack) on the mini to the line-in on the PC (usually a blue colored jack, check your PC/motherboard manual.)
Once that is in place, pull up the volume controls in XP (double click the speaker in the system tray), go to options->properties, check the "Line In" option. This enables line-in and places the input controls on your volume control panel. Now adjust your line-in volume. I left mine all the way up.
That's it, your sound card should pass the line-in sound through to your line-out.
Some instructions I found online say to get a Y-splitter and run both computers line-out to the speakers. Not only can this create unwanted line-noise, but you can easily damage the sound cards of your computers. Passing the sound through one of the computers is the safest way to go, and only requires one cable.
Once that is in place, pull up the volume controls in XP (double click the speaker in the system tray), go to options->properties, check the "Line In" option. This enables line-in and places the input controls on your volume control panel. Now adjust your line-in volume. I left mine all the way up.
That's it, your sound card should pass the line-in sound through to your line-out.
Some instructions I found online say to get a Y-splitter and run both computers line-out to the speakers. Not only can this create unwanted line-noise, but you can easily damage the sound cards of your computers. Passing the sound through one of the computers is the safest way to go, and only requires one cable.
Saturday, September 27, 2008
Enter text by swiping?
This is a very interesting idea, maybe apple could implement it?
http://www.swypeinc.com/product.html
The idea is to enter words by swiping your finger over the letters on the keyboard. You type a word by running your finger over the letters in one motion, and the phone figures out the word. It seems this would be a very quick way to type, so long as the word recognition is accurate.
http://www.swypeinc.com/product.html
The idea is to enter words by swiping your finger over the letters on the keyboard. You type a word by running your finger over the letters in one motion, and the phone figures out the word. It seems this would be a very quick way to type, so long as the word recognition is accurate.
Monday, September 15, 2008
Poker Stars for the iPhone?
Can you play Poker Stars on an iPhone? The short answer is yes, but with some setup and a bit of effort. This is a proof of concept, using the VNC viewer to display the poker table on the phone. As you can see, I had a bit of trouble trying to change my raise amount, as the pointer didn't quite line up. The VNC buttons were in the way too, but the screen could be moved. With a little effort, it could work. This requires a computer setup and running the PS client before you can view it on the phone. Obviously, a native poker app would be best. Poker Stars, are you watching?
Sunday, September 7, 2008
Buy-in for the mininum or maximum?
You sit down at the 2-5 No-Limit table. The buy-in is $200 minimum, and $500 maximum. What should you buy in for? Does it make any difference?
For a long time I thought that buying in for the max was the best choice, as this gives you an opportunity to put the most money into a hand when you hold the nuts. It makes sense. But lets look at this from another perspective.
Hypothetical situation: let's say you are heads up, you have $200 and the other guy has $800 (or more.) You both go all-in BLIND 2 hands in a row. Who comes out ahead on average?
Here are the 3 possible outcomes:
You lose the first hand, game over. He profits $200.
You win the first hand, lose the second. Game over, he profits $200.
You win the first and second hand. You profit $600.
Even though you only win 1 of 3 situations, the amount you make is $200 more than the other guy's winnings combined.
This observation alone makes a clear choice that there is an advantage to buying in short. A good strategy (online or live play) is to buy in for the minimum, and stand up and leave as soon as you double up your bankroll. There is no sense in gambling your winnings back, just leave and buy-in short again later (live), or on another table (online.)
For a long time I thought that buying in for the max was the best choice, as this gives you an opportunity to put the most money into a hand when you hold the nuts. It makes sense. But lets look at this from another perspective.
Hypothetical situation: let's say you are heads up, you have $200 and the other guy has $800 (or more.) You both go all-in BLIND 2 hands in a row. Who comes out ahead on average?
Here are the 3 possible outcomes:
You lose the first hand, game over. He profits $200.
You win the first hand, lose the second. Game over, he profits $200.
You win the first and second hand. You profit $600.
Even though you only win 1 of 3 situations, the amount you make is $200 more than the other guy's winnings combined.
This observation alone makes a clear choice that there is an advantage to buying in short. A good strategy (online or live play) is to buy in for the minimum, and stand up and leave as soon as you double up your bankroll. There is no sense in gambling your winnings back, just leave and buy-in short again later (live), or on another table (online.)
Wednesday, July 23, 2008
The days to come with Apple
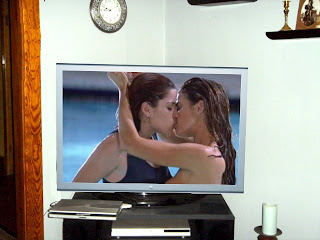
What is coming next from Apple? One can only speculate. There has been a lot of talk about a tablet computer. That makes sense, take your iPhone and blow it up to a bigger version, using the same multi-touch interface.
So let's take a step back and see what Apple has done so far, and how that can be applied to what is coming.
Apple was strictly a computer company. Then one day, they decided to take a new direction and created the iPod. This was not a traditional computer per se, but a digital music box in your pocket. Not only did Apple break away from it's normal business operations, but they encroached on the music business. They made hardware for listening to music, they created iTunes, and now control a large portion of the sale of music. Obviously, a huge success.
Then they started making other hardware gadgets to compliment the personal computer, such as wireless routers. Again, stretching their business tactics beyond the PC. Then comes AppleTV, a hardware solution to watching digital video media. Then of course, the iPhone. A cell phone from Apple. So now they directly compete with the cell phone industry.
You get the idea... the Apple technology keeps spilling over into new technologies. What is going to happen next? Here are some fun speculations:
* Apple personal communication (phone?) service. Instead of partnering with cell phone providers, they create their OWN personal communication network. Maybe this won't be cellular technology, but it will certainly directly compete with it. Maybe this will be purely online, via something like Skype? Once everyone is wired in, this will certainly be viable. Or maybe they will do something on their own frequency. Who knows?
* Apple Digital Television. No, I'm not talking about the AppleTV that hooks to your TV set, I'm talking about the TV ITSELF. We'll call it iTube. Imagine a 60" Apple branded TV in your living room. Integrated internet access, iPhone/iTablet remote controlled, jacked into your Apple network via bonjour services. Again, encroaching on the TV industry, but why not? They already make 30" monitors. This leads to...
* Apple Digital Home Theater. Maybe this will be combined with the iTube? Full digital home stereo, speakers, the works. Every component of your home theater with the Apple brand. Goodbye Onkyo, Harmon Kardon, Sony... Hello Apple! Why go through the pain of figuring out what components to buy? Just get Apple... you know it will be excellent quality, it will be compatible with your other Apple hardware, and certainly look fantastic like everything else Apple makes. Not to mention, one cable coming off the back instead of the horrendous tangle of wires in your current system.
* Apple Home Automation. Imagine controlling everything in your home with your Apple computer. Grab your iPhone and turn down the lights. Turn up the furnace. View the camera at the front door. Open the garage door. Warm up the car. Set your alarm. Preheat the oven. Re-program your sprinkler system. Listen in on any room in the house. All of this from in the living room, or from any remote location. Think of the possibilities!
* Apple Home Security. Yep, secure your home with Apple. Motion detectors can alert you from afar, you can instantly view any room in your house and notify the police. The police can then monitor with you, and know exactly what course of action to take from outside.
Now we really start thinking out of the box:
* The iCar. (My business partner and I dreamt this up, I can't take all the credit.) I can hear Steve introducing it at a keynote: "We looked at all of the existing cars out there. The wheels. The suspension. The drive train. The engine. We decided to get rid of ALL of it and start over! Introducing the iCar." A cover slips off of a very simple looking contraption, similar to an egg with a seamless door. The car is hovering over the ground. The door silently glides open and Steve hops in. "We've decided that the iCar needs to magnetically levitate. Therefore, it can only travel on iRoads. Have no fear, we are already working on an infrastructure of iRoads, to be spanning the globe over the next several years." The dash is conspicuous... just one single button. Steve gives it a press, and the entire dash lights up, like a big seamless iPhone interface.
You can use your own imagination from there. What's next? Homes? Buildings? Cities? (I'm from Apple City, USA!), world domination? You decide in the comments!
Tuesday, July 22, 2008
Reasons why people buy the iPhone
So how many blogs have written "10 reasons why the iPhone sucks." I've seen them, I've read them, they have their points. But the point they are missing, is the reasons why people DO buy the iPhone. The good things far outweigh the bad. Here is my list of the good things.
* Multi-touch. This has to be the biggest breakthrough in mobile usability. Pinch and squeeze the items to zoom in and out, flick or swipe your finger across the interface to effortlessly move through your contacts and photo albums. Nothing comes close to the response and feel of an iPhone.
* Safari. The mobile browser that really does it all. And I don't have to convince you of this, the statistics speak for themselves. Give them a web browser they can use, and they will use it. Pinch zooming, double-tap focus, finger slide panning... hands down, no other mobile browser comes close to it's usability.
* The iPod. Let's face it, Apple built the iPhone with iPod users in mind. You don't need to carry two things around, and the iPod interface on the iPhone is easiest and most feature-rich thus far. For those already tapped into the iTunes ecosystem, the iPhone is an easy choice.
* The App Store. One place to get apps, available to every iPhone. Browse through applications and install them with ease. If you have ever gone through the gauntlet of installing and maintaining apps on platforms such as Windows Mobile, you will deeply appreciate the simplicity of the app store. For developers, you don't need hosting, and you get an instant world-wide audience!
* multi-lingual. This may not apply to individual phone owners, but being a touch-screen oriented device, the available languages are limitless, which is a very good thing for Apple supplying other countries with iPhones, and app developers can write their applications for any number of languages.
* The development platform. Cocoa and OS X have rave reviews on it's easy-to-use development tools (free with OS X), and the iPhone is no exception. Quickly and easily deploy applications on the iPhone that adhere to the apple user interface guidelines.
Of course there are shortcomings of the iPhone (You can read other blogs to get the juice.) But realize, the iPhone is in its infancy. After one year, Apple has completely changed the face of the mobile industry. IMHO, there are only better things to come.
* Multi-touch. This has to be the biggest breakthrough in mobile usability. Pinch and squeeze the items to zoom in and out, flick or swipe your finger across the interface to effortlessly move through your contacts and photo albums. Nothing comes close to the response and feel of an iPhone.
* Safari. The mobile browser that really does it all. And I don't have to convince you of this, the statistics speak for themselves. Give them a web browser they can use, and they will use it. Pinch zooming, double-tap focus, finger slide panning... hands down, no other mobile browser comes close to it's usability.
* The iPod. Let's face it, Apple built the iPhone with iPod users in mind. You don't need to carry two things around, and the iPod interface on the iPhone is easiest and most feature-rich thus far. For those already tapped into the iTunes ecosystem, the iPhone is an easy choice.
* The App Store. One place to get apps, available to every iPhone. Browse through applications and install them with ease. If you have ever gone through the gauntlet of installing and maintaining apps on platforms such as Windows Mobile, you will deeply appreciate the simplicity of the app store. For developers, you don't need hosting, and you get an instant world-wide audience!
* multi-lingual. This may not apply to individual phone owners, but being a touch-screen oriented device, the available languages are limitless, which is a very good thing for Apple supplying other countries with iPhones, and app developers can write their applications for any number of languages.
* The development platform. Cocoa and OS X have rave reviews on it's easy-to-use development tools (free with OS X), and the iPhone is no exception. Quickly and easily deploy applications on the iPhone that adhere to the apple user interface guidelines.
Of course there are shortcomings of the iPhone (You can read other blogs to get the juice.) But realize, the iPhone is in its infancy. After one year, Apple has completely changed the face of the mobile industry. IMHO, there are only better things to come.
Tuesday, June 3, 2008
Setup Thunderbird 2.0 for Gmail
I thought I'd share how I setup Thunderbird 2.0 to handle Gmail accounts so the folders match up. First follow the install instructions from the Gmail website for Thunderbird 2.0. Then, apply the following:
*) Go to Account Settings -> Server Settings
*) in the box that says Server Settings, click Advanced...
*) For the IMAP Server Directory, type [Gmail] (with the brackets)
*) click OK
*) Go Account Settings -> Copies & Folders
*) check Place a copy in:
*) select the Other: radio button
*) select the Sent Mail folder under your Gmail account in the dropdown
*) Repeat the above process for Drafts & Templates, choosing the Drafts folder on Gmail
*) Go to Account Settings -> Junk Settings
*) Repeat the above process for the Junk folder, choosing the Spam folder on Gmail
Thats it! You will no longer see a [Gmail] subfolder, and your Sent, Drafts, and Spam folders will match up to the correct Gmail folders.
*) Go to Account Settings -> Server Settings
*) in the box that says Server Settings, click Advanced...
*) For the IMAP Server Directory, type [Gmail] (with the brackets)
*) click OK
*) Go Account Settings -> Copies & Folders
*) check Place a copy in:
*) select the Other: radio button
*) select the Sent Mail folder under your Gmail account in the dropdown
*) Repeat the above process for Drafts & Templates, choosing the Drafts folder on Gmail
*) Go to Account Settings -> Junk Settings
*) Repeat the above process for the Junk folder, choosing the Spam folder on Gmail
Thats it! You will no longer see a [Gmail] subfolder, and your Sent, Drafts, and Spam folders will match up to the correct Gmail folders.
Thursday, May 29, 2008
Winning No Limit Hold-Em
It's been awhile since I've played some live poker, but I went to the casino an hour up the road over the weekend. I turned $100 into $850 at the $1-3 NL table in about six hours. Not bad! Although I'll have to admit, there were some frustrated people at the table, and I'll tell you why.
To several of the players, I seemingly played junk cards most of the time, and took down some very large pots with them. I can see how this can be frustrating when you have solid preflop cards, raise with them, get called and get taken by a lucky flop. But there is a method to the madness, and I'll try to explain.
First of all, I like to show down a big bluff somewhere in the beginning of my game. I'll raise preflop with junk, bet the flop, bet the turn, bet the river and turn up next to nothing. Now they have a perception of a loose foolish player at the table. It works even better if I get lucky and hit two pair or something. I just make sure my betting made no sense. I'll do this now and then, especially if the table is changing players often. I like to keep the loose image. This helps my strong hands make more, and also keeps them guessing.
Now, in my observation of the game of No-Limit (especially at these smaller stakes,) cards like pocket aces or pocket kings will typically win a small pot or lose a large one. On the other hand, cards like small suited connectors, small pairs, small one gappers are the opposite: they will typically lose a small pot, or win a large one.
One reason is the difference in starting strength of the hand. If pocket aces gets no help on the flop, it's going to be hard to fold that hand in any event. Whereas something like 56, if the flop isn't strong, it's an easy fold.
Another is deception. You call preflop with 56 and the flop comes 56T rainbow, the aces aren't going to be too concerned about losing with that texture of a flop and they'll likely pay you off nicely. Whereas the flop comes 9TJ, the aces will be a bit more cautious if they get re-raised.
Holding small cards, the flop that helps you is not likely going to be helping another player in the hand. When you call a preflop raise, you are typically going to be up against stronger starting hands, and that helps when you hit the flop right. You don't have to guess if you have 2nd best, such as a hand like TJ might be doing with a flop of TJQ.
If you are going to try playing this style, remember implied odds, that is the most important thing. That is, if you are going to call a preflop raise with little cards, make sure you have a bankroll to back it up, as well as the guy you are calling. You want sufficient implied odds so when you hit the flop, you get paid off.
To several of the players, I seemingly played junk cards most of the time, and took down some very large pots with them. I can see how this can be frustrating when you have solid preflop cards, raise with them, get called and get taken by a lucky flop. But there is a method to the madness, and I'll try to explain.
First of all, I like to show down a big bluff somewhere in the beginning of my game. I'll raise preflop with junk, bet the flop, bet the turn, bet the river and turn up next to nothing. Now they have a perception of a loose foolish player at the table. It works even better if I get lucky and hit two pair or something. I just make sure my betting made no sense. I'll do this now and then, especially if the table is changing players often. I like to keep the loose image. This helps my strong hands make more, and also keeps them guessing.
Now, in my observation of the game of No-Limit (especially at these smaller stakes,) cards like pocket aces or pocket kings will typically win a small pot or lose a large one. On the other hand, cards like small suited connectors, small pairs, small one gappers are the opposite: they will typically lose a small pot, or win a large one.
One reason is the difference in starting strength of the hand. If pocket aces gets no help on the flop, it's going to be hard to fold that hand in any event. Whereas something like 56, if the flop isn't strong, it's an easy fold.
Another is deception. You call preflop with 56 and the flop comes 56T rainbow, the aces aren't going to be too concerned about losing with that texture of a flop and they'll likely pay you off nicely. Whereas the flop comes 9TJ, the aces will be a bit more cautious if they get re-raised.
Holding small cards, the flop that helps you is not likely going to be helping another player in the hand. When you call a preflop raise, you are typically going to be up against stronger starting hands, and that helps when you hit the flop right. You don't have to guess if you have 2nd best, such as a hand like TJ might be doing with a flop of TJQ.
If you are going to try playing this style, remember implied odds, that is the most important thing. That is, if you are going to call a preflop raise with little cards, make sure you have a bankroll to back it up, as well as the guy you are calling. You want sufficient implied odds so when you hit the flop, you get paid off.
Thursday, April 17, 2008
Poker Tells Database
Poker tells are one of the most fascinating an fun elements of poker. It's always great to hear about a new tell that someone has examined on a player. However, there is no convenient place to browse through poker tells and contribute your own. So, I built one.
www.pokertellsdb.com is an online poker tells database, where anyone can contribute poker tells, as well as rate and comment on them. I'll consider this in "beta" since I built the entire system in the course of a couple of days. Let me know what you think, and what features it could use. It's fairly sparse since I only added a few tells I could think of right off hand. Please contribute!
http://www.pokertellsdb.com/
www.pokertellsdb.com is an online poker tells database, where anyone can contribute poker tells, as well as rate and comment on them. I'll consider this in "beta" since I built the entire system in the course of a couple of days. Let me know what you think, and what features it could use. It's fairly sparse since I only added a few tells I could think of right off hand. Please contribute!
http://www.pokertellsdb.com/
Tuesday, February 5, 2008
Playing Pocket Aces
Sitting at the local poker room, you take a look at your hole cards and see pocket aces. A rush goes through your body. Now, what is the best course of action? How do you maximize your profit, and minimize your bad beats?
Generally speaking, raise with them. Raise and re-raise. If you are first to act, a good starting point is roughly 3-4 times the big blind. If you are behind a raiser, raise 2-3 times what he raised. The key here is to minimize callers. You don't want more than one or two. On the flop, no matter what comes out, put out a continuation bet. I like to mix it up from 75-100% of the pot. Usually you can take the hand down right here. If you get called or re-raised, then it is time to analyze your position, your opponent and stack sizes. Usually you aren't going to get out of the hand unless you are really sure you are beat. If your opponent out-flopped you then that is the breaks. But, the important thing is that you make them pay to see the flop. This will keep your aces on the winning side in the long run.
Some like to slow-play the aces. Although it is nice to mix up your play, typically you will have a chance at more money by raising. Let's take an example, and play the hand two different ways.
Let's say you hold aces, and a player across the table holds queens. You limp in with hopes that someone behind you will raise. The queens raise 3xbb, and you re-raise to 3x that amount. Now you have the queens suspicious. Your hand looks very strong from an early position check-raise, and they are right! You've basically given away the strength of your hand, and now the astute player may fold the queens right here.
Now lets rewind. This time we raise with our aces 4xbb. The queens re-raise 3x your raise. Now your re-raise again 2-3x his raise. At this point the queens have a LOT more money in the middle before they have determined the strength of your hand. Many times they will be committed, and call your raise, or move all-in. This is exactly the situation you want with your aces.
Regarding this whole raising strategy, it applies to most all of your poker play. Look for reasons to raise, and keep check-raises to a minimum. If you flop a straight, raise. If you flop a set, come out betting! You are likely to get more money out of those hands betting/raising than check-raising. Raising is a big part of winning poker, so don't miss that opportunity. About the only time you don't want to raise is if you have the board completely crippled, such as flopping quads or the nut boat. In that case, you may want to check and give your opponents a chance to catch a card or bluff at you.
Generally speaking, raise with them. Raise and re-raise. If you are first to act, a good starting point is roughly 3-4 times the big blind. If you are behind a raiser, raise 2-3 times what he raised. The key here is to minimize callers. You don't want more than one or two. On the flop, no matter what comes out, put out a continuation bet. I like to mix it up from 75-100% of the pot. Usually you can take the hand down right here. If you get called or re-raised, then it is time to analyze your position, your opponent and stack sizes. Usually you aren't going to get out of the hand unless you are really sure you are beat. If your opponent out-flopped you then that is the breaks. But, the important thing is that you make them pay to see the flop. This will keep your aces on the winning side in the long run.
Some like to slow-play the aces. Although it is nice to mix up your play, typically you will have a chance at more money by raising. Let's take an example, and play the hand two different ways.
Let's say you hold aces, and a player across the table holds queens. You limp in with hopes that someone behind you will raise. The queens raise 3xbb, and you re-raise to 3x that amount. Now you have the queens suspicious. Your hand looks very strong from an early position check-raise, and they are right! You've basically given away the strength of your hand, and now the astute player may fold the queens right here.
Now lets rewind. This time we raise with our aces 4xbb. The queens re-raise 3x your raise. Now your re-raise again 2-3x his raise. At this point the queens have a LOT more money in the middle before they have determined the strength of your hand. Many times they will be committed, and call your raise, or move all-in. This is exactly the situation you want with your aces.
Regarding this whole raising strategy, it applies to most all of your poker play. Look for reasons to raise, and keep check-raises to a minimum. If you flop a straight, raise. If you flop a set, come out betting! You are likely to get more money out of those hands betting/raising than check-raising. Raising is a big part of winning poker, so don't miss that opportunity. About the only time you don't want to raise is if you have the board completely crippled, such as flopping quads or the nut boat. In that case, you may want to check and give your opponents a chance to catch a card or bluff at you.
Tuesday, January 22, 2008
The Truth about Slot Machines
This is a follow-up to my blog post about KENO playing. There are many misconceptions about how slot machines work, and I'd like to clear them up. Some people that play slots tend to think a slot machine is "hot", or "due to hit soon" because it hasn't hit in awhile. Hopefully I can make it clear why that isn't so.
Firstly, a slot machine works on pure probability. Every spin has the same chance to win. Let's say we have a slot machine that has a 100,000 in 1 chance to hit the jackpot. That means that every time you spin the reels, you have a 100,000 in 1 chance of hitting the jackpot. So what happens if you spin the reels exactly 100,000 times in a row? Will the jackpot hit once somewhere in those spins? The odds predict this, but it's not necessarily so. It might hit once, or it might not hit at all. Or, it might hit several times. You might hit two in a row! Or it might go a million pulls in a row without ever hitting one jackpot.
Since every pull has an equal chance to win, they are completely independent of each other. That means, if the slot hasn't hit in 90,000 consecutive spins, that does NOT mean it has 10,000 spins left before the jackpot hits. The last 90,000 spins have zero influence on what is yet to come. You can pretty much figure that any time you sit down at this slot machine, it will probably hit the jackpot sometime in the next 100,000 spins, regardless of what has happened in the past.
If you are playing on a slot machine with a progressive jackpot, you can keep a rough estimate on how long it has been since it hit. The higher the progressive, the longer it has been since the last time it hit. But, your odds of winning never change. Whether the jackpot just hit, or it has been a year since it hit, your chances of winning on each spin of the reel are exactly the same.
Some say they've never seen a jackpot hit early, but it does hit a very short time after the progressive gets very big. Why is that?
Well, if the jackpot hit very early, you probably wouldn't even notice. Say the jackpot hits and starts over at $1,000. You come back a week later at the jackpot has progressed to $1800. What you don't realize is that the jackpot may have hit again at $1200 and reset to $1000, then climbed it's way back to $1800. When the jackpot hits early, you can't really see any consequences of it.
So why does it seem to hit soon after it gets very big? It's a matter of sheer numbers: the more spins that happen, the more chances there are to hit the jackpot. Every single spin of the reels still has a 100,000 to 1 chance to hit, but if the slot gets a whopping 100,000 spins in one week, it is likely that the jackpot will hit sometime during that week. But again, it might not hit at all, or might hit several times in that week. After the jackpot is hit, the volume of spins will most certainly die down to a trickle again, at least until it builds up again.
Now you might be inclined to play the slot machine with the big progressive, and that makes sense: if you are lucky enough to hit the jackpot, it pays out better than a machine that hit recently with low progressive. But your odds of winning are no different than playing a slot machine that hit one minute ago.
This logic can be carried over to the roulette wheel. Casino's have started posting the recent numbers that have hit. But, those numbers have zero influence on future spins of the reel. You can play the same number all night, or switch numbers every spin, it really doesn't matter.
Keno is very much the same. The numbers that have hit recently have no influence on the upcoming numbers. You can play the same numbers all night, or switch numbers every ticket. It doesn't make a bit of difference in your chances to win.
Firstly, a slot machine works on pure probability. Every spin has the same chance to win. Let's say we have a slot machine that has a 100,000 in 1 chance to hit the jackpot. That means that every time you spin the reels, you have a 100,000 in 1 chance of hitting the jackpot. So what happens if you spin the reels exactly 100,000 times in a row? Will the jackpot hit once somewhere in those spins? The odds predict this, but it's not necessarily so. It might hit once, or it might not hit at all. Or, it might hit several times. You might hit two in a row! Or it might go a million pulls in a row without ever hitting one jackpot.
Since every pull has an equal chance to win, they are completely independent of each other. That means, if the slot hasn't hit in 90,000 consecutive spins, that does NOT mean it has 10,000 spins left before the jackpot hits. The last 90,000 spins have zero influence on what is yet to come. You can pretty much figure that any time you sit down at this slot machine, it will probably hit the jackpot sometime in the next 100,000 spins, regardless of what has happened in the past.
If you are playing on a slot machine with a progressive jackpot, you can keep a rough estimate on how long it has been since it hit. The higher the progressive, the longer it has been since the last time it hit. But, your odds of winning never change. Whether the jackpot just hit, or it has been a year since it hit, your chances of winning on each spin of the reel are exactly the same.
Some say they've never seen a jackpot hit early, but it does hit a very short time after the progressive gets very big. Why is that?
Well, if the jackpot hit very early, you probably wouldn't even notice. Say the jackpot hits and starts over at $1,000. You come back a week later at the jackpot has progressed to $1800. What you don't realize is that the jackpot may have hit again at $1200 and reset to $1000, then climbed it's way back to $1800. When the jackpot hits early, you can't really see any consequences of it.
So why does it seem to hit soon after it gets very big? It's a matter of sheer numbers: the more spins that happen, the more chances there are to hit the jackpot. Every single spin of the reels still has a 100,000 to 1 chance to hit, but if the slot gets a whopping 100,000 spins in one week, it is likely that the jackpot will hit sometime during that week. But again, it might not hit at all, or might hit several times in that week. After the jackpot is hit, the volume of spins will most certainly die down to a trickle again, at least until it builds up again.
Now you might be inclined to play the slot machine with the big progressive, and that makes sense: if you are lucky enough to hit the jackpot, it pays out better than a machine that hit recently with low progressive. But your odds of winning are no different than playing a slot machine that hit one minute ago.
This logic can be carried over to the roulette wheel. Casino's have started posting the recent numbers that have hit. But, those numbers have zero influence on future spins of the reel. You can play the same number all night, or switch numbers every spin, it really doesn't matter.
Keno is very much the same. The numbers that have hit recently have no influence on the upcoming numbers. You can play the same numbers all night, or switch numbers every ticket. It doesn't make a bit of difference in your chances to win.
Subscribe to:
Posts (Atom)